Handling Authentication and Authorization in Node.js
Introduction
In today’s world of web and mobile applications, ensuring secure access to resources is a critical requirement. Developers must handle authentication (verifying the identity of users) and authorization (determining what resources a user can access) effectively to maintain the integrity and security of their systems. Node.js, with its non-blocking, event-driven architecture, is a popular platform for building scalable web applications, and handling secure user authentication is a key part of that.
In this article, we’ll dive into how authentication and authorization work in Node.js, exploring different methods and best practices to help you build secure, reliable applications.
What is Authentication?
Authentication is the process of verifying a user’s identity. It typically involves users providing credentials (such as a username and password) and the server confirming whether those credentials are valid.
There are multiple ways to implement authentication in Node.js, with common methods including:
- Session-based Authentication
- Token-based Authentication (e.g., JWT)
- OAuth and Social Logins
What is Authorization?
Authorization defines what actions an authenticated user is allowed to perform within an application. After a user’s identity is verified, the application must determine which resources or functionalities the user can access.
Authorization typically comes into play after authentication, once the user’s identity is known. Examples of authorization include:
- Allowing users to view only their own data
- Restricting admin-only actions to users with specific roles
Popular Authentication Methods in Node.js
1. Session-Based Authentication
Session-based authentication is one of the oldest and most widely used methods. In this approach, after a user successfully logs in, the server creates a session and stores the session ID on the server. The session ID is then sent to the client (browser) via cookies. For each subsequent request, the browser sends this cookie, and the server verifies the session ID to authenticate the user.
Steps for Session-Based Authentication:
- User submits credentials (username, password).
- Server verifies the credentials.
- If valid, a session is created, and the session ID is stored in the server.
- The session ID is sent to the client as a cookie.
- For future requests, the client sends the session ID, and the server uses it to identify the user.
Pros:
- Well-established and supported by many libraries, such as express-session.
- Server-side session management allows for easy session invalidation.
Cons:
- Scalability issues with server-side session storage.
- Not ideal for stateless architectures or APIs.
Example Using express-session:
const express = require('express'); const session = require('express-session'); const app = express(); app.use(session({ secret: 'your-secret-key', resave: false, saveUninitialized: true, cookie: { secure: false } // Set to true in production })); app.post('/login', (req, res) => { // Authenticate user here req.session.userId = user.id; // Save userId in session res.send('Logged in!'); });
2. Token-Based Authentication (JWT)
JWT (JSON Web Tokens) is a stateless authentication method that has become very popular for modern web applications, especially single-page applications (SPAs) and APIs. With JWT, the server generates a token (usually signed) that contains a payload with user information. This token is then sent to the client, which stores it (typically in localStorage or sessionStorage).
For subsequent requests, the client sends the token in the Authorization header. The server then verifies the token to authenticate the user.
Steps for JWT Authentication:
- User submits credentials.
- Server verifies the credentials.
- If valid, the server generates a JWT containing user information.
- The client stores the JWT and sends it in the Authorization header for future requests.
- The server verifies the JWT and authenticates the user.
Pros:
- JWTs are stateless, so no session management is required on the server.
- Ideal for APIs and microservices.
- Easy to scale since the server doesn’t need to store session data.
Cons:
- Once issued, the server cannot easily invalidate a JWT unless additional mechanisms (e.g., blacklists) are used.
- Sensitive to client-side storage vulnerabilities.
Example Using jsonwebtoken:
const jwt = require('jsonwebtoken'); const express = require('express'); const app = express(); // Secret key const SECRET_KEY = 'your-secret-key'; app.post('/login', (req, res) => { const user = authenticateUser(req.body); // Custom function to verify credentials if (user) { const token = jwt.sign({ userId: user.id }, SECRET_KEY, { expiresIn: '1h' }); res.json({ token }); } else { res.status(401).send('Invalid credentials'); } }); app.get('/protected', (req, res) => { const token = req.headers['authorization']; if (token) { jwt.verify(token, SECRET_KEY, (err, decoded) => { if (err) { return res.status(403).send('Invalid token'); } req.userId = decoded.userId; res.send('Protected content'); }); } else { res.status(401).send('No token provided'); } });
3. OAuth and Social Logins
OAuth allows users to authenticate through third-party services like Google, Facebook, or GitHub without needing to create new credentials for your application. This is commonly referred to as “social login.”
Using OAuth 2.0, you can redirect users to the provider’s login page. Once authenticated, the provider sends a token that your server can use to identify the user and grant access.
Steps for OAuth 2.0:
- The user clicks on a “Login with Google” button (for example).
- The user is redirected to the Google login page.
- After logging in, Google sends a token back to your server.
- Your server uses the token to request user information and authenticate them.
Pros:
- Simplifies the login process for users.
- No need to manage sensitive information like passwords.
- Reduces friction, encouraging higher user registration rates.
Cons:
- Reliant on third-party services.
- Requires integration with OAuth providers and proper handling of tokens.
Example Using passport.js with Google OAuth:
const passport = require('passport'); const GoogleStrategy = require('passport-google-oauth20').Strategy; passport.use(new GoogleStrategy({ clientID: 'GOOGLE_CLIENT_ID', clientSecret: 'GOOGLE_CLIENT_SECRET', callbackURL: '/auth/google/callback' }, (token, tokenSecret, profile, done) => { // Save user information here return done(null, profile); })); app.get('/auth/google', passport.authenticate('google', { scope: ['profile'] })); app.get('/auth/google/callback', passport.authenticate('google', { failureRedirect: '/' }), (req, res) => { res.redirect('/dashboard'); } );
Role-Based Authorization
Once users are authenticated, you need to manage what they can and cannot do based on their roles. For example, you might have roles like admin, editor, and user, each with different access levels.
Example of Role-Based Authorization:
function authorize(roles = []) { return (req, res, next) => { const user = req.user; // Assume user info is added to req after authentication if (roles.length && !roles.includes(user.role)) { return res.status(403).json({ message: 'Access denied' }); } next(); }; } app.get('/admin', authorize(['admin']), (req, res) => { res.send('Admin content'); });
Best Practices for Authentication and Authorization in Node.js
- Use HTTPS: Always secure your Node.js application with SSL/TLS to protect user data.
- Encrypt Sensitive Data: Never store passwords in plaintext. Use hashing algorithms like bcrypt for password storage.
- Token Expiry: Set expiration times for JWTs to minimize the risk of token misuse.
- Session Security: If using sessions, implement proper session management practices, such as limiting session lifetimes and regenerating session IDs on login.
- Use Security Libraries: Libraries like Helmet and csurf help protect against common web vulnerabilities like CSRF and XSS.
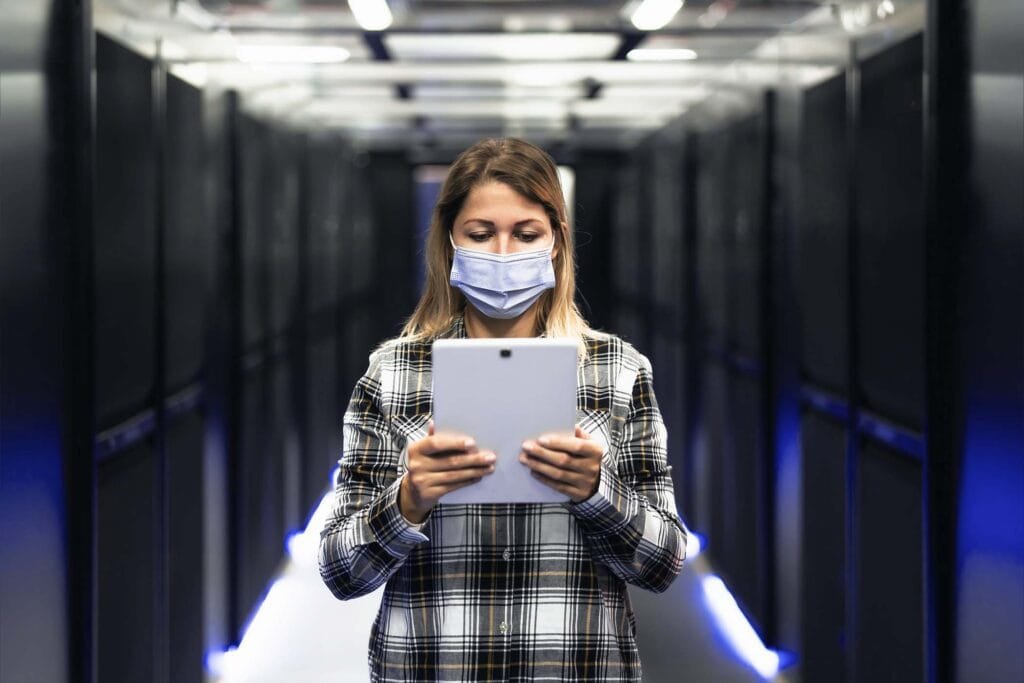
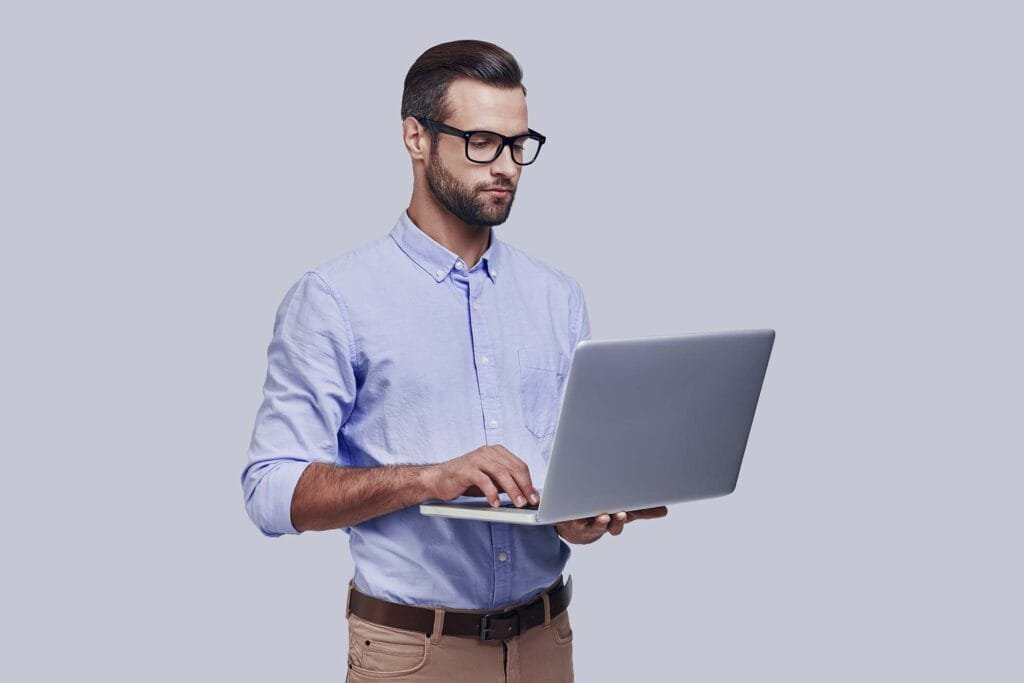
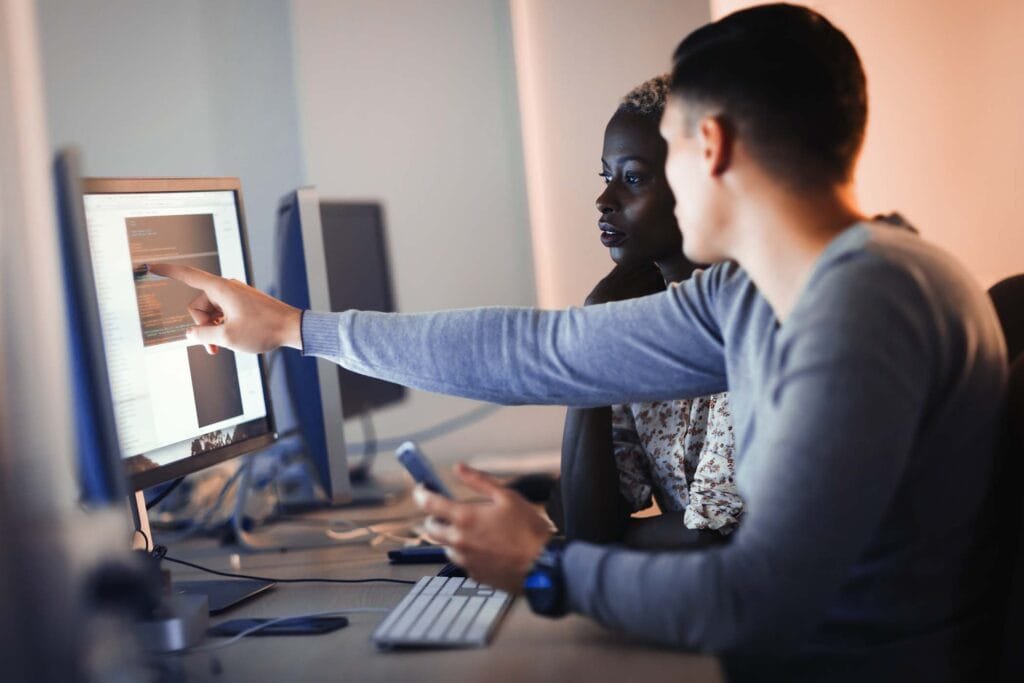
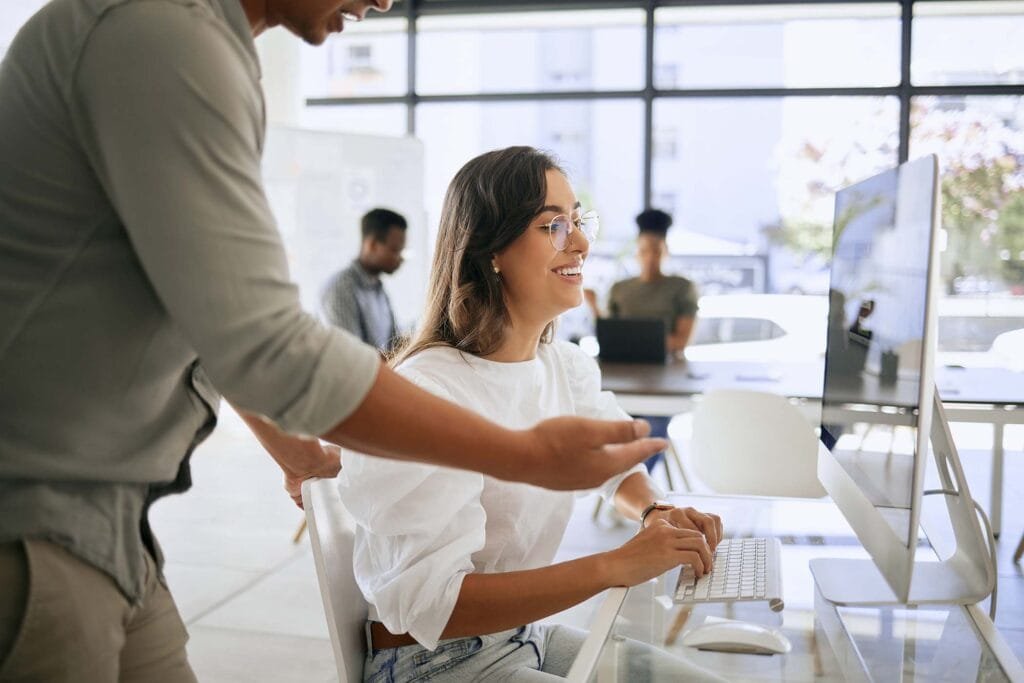
Conclusion
Handling authentication and authorization in Node.js is a fundamental aspect of securing your applications. Whether you’re building session-based login systems, using JWT for stateless authentication, or integrating OAuth for social logins, Node.js provides powerful tools and libraries to streamline these processes.
By understanding the differences between various authentication methods and following best practices, you can ensure that your Node.js applications remain secure, scalable, and user-friendly.
Post Tags :
Share :